Imagine you’re building a new software product for your company. You want it to be scalable, easy to maintain, and future-proof. But when it comes to choosing the right programming paradigm, the decision isn’t always straightforward. What should you go with—functional programming vs OOP?
Many businesses stick to what they know—OOP, the traditional approach that organizes code into objects. But functional programming vs OOP is a debate that’s growing louder, especially as FP gains traction in areas like big data, machine learning, and cloud computing.
So, how do you decide? Let’s break down the key differences, advantages, and business implications to help you make an informed, strategic choice.
Want to create stunning, high-performance interfaces? Elevate your UX with our frontend experts today!
Functional Programming—A Comprehensive Overview
Functional Programming (FP) is a declarative programming paradigm that treats computation as the evaluation of mathematical functions. Unlike Object-Oriented Programming (OOP), which organizes code into objects with states and behaviors, FP focuses on writing pure functions and avoiding shared state or mutable data.
FP is widely used in areas like data processing, cloud computing, and AI-driven applications because of its efficiency, scalability, and reliability. Let’s break it down step by step to understand how it works and why businesses are increasingly adopting it.
Core Principles of Functional Programming
FP is based on a few key principles that define its structure and approach to problem-solving. These principles make functional programming vs OOP fundamentally different and are essential to understanding why some organizations prefer this paradigm.
1. Pure Functions
A pure function is a function that:
- Always returns the same output for the same input and has no hidden state.
- Has no side effects, meaning it doesn’t modify global variables or external data.
Example of a Pure Function in Python
def add(x, y):
return x + y
- The function add(2, 3) will always return 5, no matter when or where it’s called.
Why Do Pure Functions Matter?
- Debugging becomes easier. Since functions don’t depend on hidden variables, tracking errors is simple.
- Code becomes more reusable as functions work independently; they can be used across multiple applications.
- Parallel execution is efficient. No shared state means functions can run simultaneously without interference.
In contrast, impure functions might rely on external variables, which can make debugging and testing difficult.
Example of an Impure Function
total = 0
def add_to_total(x):
global total
total += x
return total
- Since the total is a global variable, calling add_to_total(5) multiple times returns different results, making debugging and predicting behavior harder.
2. Immutability
Immutability means that data cannot be changed once created. Instead of modifying existing values, FP creates new data structures whenever an update is needed.
Example of an Immutable Data in Python
old_list = [1, 2, 3]
new_list = old_list + [4] # A new list is created instead of modifying old_list
- The original old_list remains unchanged, ensuring predictability and avoiding unexpected bugs.
Why Does Immutability Matter?
- It prevents accidental data modifications and reduces the risk of unintended changes affecting program behavior.
- It improves concurrency, i.e., multiple functions can access the same data without conflicts.
- It enhances maintainability. Immutable data structures make debugging easier and reduce the likelihood of errors.
Many programming languages, such as Haskell, Scala, and Clojure, enforce immutability by default, while languages like Python and JavaScript support immutable programming practices.
3. First-Class and Higher-Order Functions
FP treats functions as first-class citizens, meaning:
- Functions can be assigned to variables.
- Functions can be passed as arguments.
- Functions can return other functions.
A higher-order function is a function that either accepts another function as an argument or returns a function as output.
Example of a Higher-Order Function
def multiplier(n):
return lambda x: x * n # Returns a function
double = multiplier(2)
print(double(5)) # Output: 10
Here, multiplier(2) returns a new function that multiplies its input by 2.
Why Does Higher-Order Function Matter?
- It encourages modularity and reusability. Functions can be easily composed.
- It simplifies complex logic; instead of writing loops and conditionals, you can use pre-defined behaviors.
- It enables dynamic function creation, which is useful for AI, machine learning, and data processing.
Higher-order functions are extensively used in functional libraries like lodash.js (JavaScript) and functools (Python).
4. Recursion Over Loops
Instead of using traditional for and while loops, FP relies on recursion. Recursion is when a function calls itself until a base condition is met.
Example of a Recursion for Factorial Calculation
def factorial(n):
if n == 1:
return 1
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
- Recursion eliminates mutable loop variables and fits well with FP’s immutable nature.
Why Recursion Matters?
- It simplifies loop-based operations and is ideal for working with nested or hierarchical data (e.g., file systems).
- It works well with immutable data, as recursion avoids modifying variables.
- It is optimized in functional languages. Some languages offer tail-call optimization, which prevents excessive memory usage.
5. Declarative Programming
FP emphasizes declarative programming, meaning developers focus on what needs to be done rather than how to do it.
Example of Functional Declarative Code
numbers = [1, 2, 3, 4, 5]
evens = list(filter(lambda x: x % 2 == 0, numbers))
- This clearly states what we want (filter even numbers) without detailing how it should be done.
Why Does Declarative Programming Matter?
- It simplifies complex logic and avoids lengthy loops and conditions.
- It enhances readability, and the code is more intuitive and easier to maintain.
- It reduces bugs as there is less room for human error.
Many modern frameworks and libraries (e.g., React.js, Pandas) encourage declarative programming because it leads to cleaner and more efficient code.
Benefits of Functional Programming
FP isn’t just a theoretical concept; it has real business applications, especially in high-performance, data-driven systems. Let’s take a look at its benefits below:
- It has better code maintainability as ‘pure functions’ and ‘immutability’ reduce debugging time in functional programming.
- It supports scalability for large applications and is ideal for cloud-based and distributed systems.
- There are fewer bugs and higher code reliability in functional programming as its predictable code ensures fewer errors.
- It has improved parallel processing as immutable data and stateless functions enable seamless concurrency.
According to a study by ThoughtWorks, functional programming adoption in enterprise software has increased by 40% over the last decade. Major companies like Netflix, Twitter, and LinkedIn use FP to handle their large-scale operations.
Unlock JavaScript’s true potential—dive into functional programming and write cleaner, smarter code!
Object-Oriented Programming (OOP) — A Comprehensive Overview
Object-Oriented Programming (OOP) is one of the most widely used programming paradigms in enterprise software, large-scale applications, and interactive systems. Unlike Functional Programming (FP), which focuses on pure functions and immutability, OOP structures software around objects—bundles of data and methods that operate on that data.
OOP has been the dominant paradigm for decades and is the foundation of many programming languages, including Java, C++, Python, and C#. Businesses favor OOP because of its modularity, reusability, and ability to handle complex business logic.
Let’s explore the core principles, benefits, and real-world applications of OOP to understand why it remains a preferred choice for software development.
Core Principles of Object-Oriented Programming
OOP is structured around four fundamental principles that help your developers organize, manage, and scale software efficiently. Let’s understand the core principles of OOP below:
1. Encapsulation
Encapsulation is the concept of hiding data and restricting direct access to it. In OOP, objects bundle data (attributes) and behavior (methods) together, preventing external code from modifying internal object states directly.
Example of Encapsulation in Python
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute (cannot be accessed directly)
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance # Controlled access through a method
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
In this example:
- The balance is private (__balance), meaning it cannot be modified directly from outside the class.
- Users can only change or retrieve data through specific methods (deposit() and get_balance()).
Why Encapsulation Matters?
- It prevents unauthorized access to sensitive data (e.g., banking, authentication systems).
- It improves security by controlling how data is modified.
- It reduces complexity by keeping implementation details hidden.
Encapsulation makes OOP particularly useful in enterprise applications, financial software, and secure transactions.
2. Inheritance
Inheritance allows developers to create new classes from existing ones, promoting code reuse and reducing duplication.
Example of Inheritance in Python
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
return "I make a sound"
class Dog(Animal): # Dog class inherits from Animal
def speak(self):
return "Bark!"
dog = Dog("Buddy")
print(dog.speak()) # Output: Bark!
Here, the Dog class inherits properties from Animal, reducing the need to write the same code multiple times.
Why Does Inheritance Matter?
- It reduces code duplication and simplifies maintenance.
- It encourages hierarchical class structures (e.g., vehicle → car → electric car).
- It is useful for large-scale applications that require modularity and reusability.
Inheritance is widely used in web applications, frameworks, and APIs where reusable components are essential.
3. Polymorphism
Polymorphism allows different classes to share the same interface while implementing different behaviors.
Example of Polymorphism in Python
class Bird:
def fly(self):
return "Some birds can fly"
class Sparrow(Bird):
def fly(self):
return "Sparrow can fly"
class Penguin(Bird):
def fly(self):
return "Penguins can't fly"
birds = [Sparrow(), Penguin()]
for bird in birds:
print(bird.fly())
# Output:
# Sparrow can fly
# Penguins can't fly
- Here, different classes implement the same method (fly()) differently, allowing flexible code execution.
Why Does Polymorphism Matter?
- It simplifies complex systems by allowing flexible object interactions.
- Polymorphism enhances scalability by enabling generic code structures.
- It is commonly used in UI frameworks, game development, and automation.
Polymorphism plays a significant role in software frameworks, database management systems, and API design.
4. Abstraction
Abstraction allows developers to hide complex implementation details and expose only essential features.
Example of Abstraction in Python
from abc import ABC, abstractmethod
class Vehicle(ABC):
@abstractmethod
def move(self):
pass # Method must be implemented by subclasses
class Car(Vehicle):
def move(self):
return "Car moves on roads"
class Airplane(Vehicle):
def move(self):
return "Airplane flies in the sky"
vehicles = [Car(), Airplane()]
for vehicle in vehicles:
print(vehicle.move())
# Output:
# Car moves on roads
# Airplane flies in the sky
- Here, the Vehicle class is abstract, ensuring that all subclasses must implement the move() method.
Why Does Abstraction Matter?
- It reduces complexity by hiding unnecessary details from users.
- It encourages modular code, making large applications easier to manage.
- Abstraction is widely used in API development, database systems, and backend architecture.
Abstraction makes OOP highly suitable for business applications, SaaS platforms, and multi-layered software architectures.
Benefits of Object-Oriented Programming
OOP remains dominant in enterprise software development due to its structured approach, reusability, and scalability. Let’s see why businesses continue to rely on OOP and how they benefit from them.
- It supports efficient code management. Modular structures make large projects easier to maintain.
- It supports enhanced security as encapsulation protects sensitive data.
- It provides scalability for large applications as its inheritance and polymorphism feature allow seamless code expansion.
- It is ideal for real-world modeling as OOP structures closely resemble real-world objects and behaviors.
- It is widely adopted in many enterprise applications, from banking software to CRM tools, that are built with OOP.
According to a report by RedMonk, over 80% of enterprise applications still rely on OOP-based languages, highlighting its continued dominance.
Explore the top OOP languages that are shaping 2025 software development. Discover the best fit for your next big project!
Functional Programming vs OOP: A Comparative Analysis
Understanding the difference between functional programming vs OOP is crucial for making informed decisions in software development. While both aim to create efficient and scalable applications, their approaches, structures, and real-world applications differ significantly.
Below, we have broken down the key differences between functional programming vs OOP.
Feature | Functional Programming | OOP |
Code Structure | It is composed of pure functions | It is organized into objects |
State Management | It has an immutable state, i.e., data never changes | It has a mutable state, i.e., data is modifiable |
Reusability | It uses high-order functions | It uses inheritance and polymorphism |
Concurrency | It is optimized for multi-threading | It is harder due to the shared state |
Security | It is more secure due to immutability | It is prone to state-related bugs |
Performance | It is efficient for data processing and cloud apps | It is efficient for UI-heavy apps |
Best Use Cases | AI, big data, finance, cloud computing | UI-driven apps, games, ERP, CRM |
1. Programming Approach
FP (Functional Programming)
FP focuses on writing pure functions that do not modify data and have no side effects. Instead of objects, functions take inputs and return outputs without changing the global state.
Example of FP in Python:
def start_engine(brand, model):
return f"{brand} {model}'s engine started."
print(start_engine("Tesla", "Model S")) # Output: Tesla Model S's engine started.
- This function doesn’t rely on any stored state, making it predictable and easier to test.
- FP relies on breaking down problems into small, reusable functions instead of managing objects.
OOP (Object-Oriented Programming)
OOP, on the other hand, is based on the concept of objects—self-contained units that store data (attributes) and behaviors (methods). These objects interact with one another to perform tasks, making it easier to model real-world entities in software.
Example of OOP in Python:
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def start_engine(self):
return f"{self.brand} {self.model}'s engine started."
my_car = Car("Tesla", "Model S")
print(my_car.start_engine()) # Output: Tesla Model S's engine started.
- Here, the Car class acts as a blueprint for creating objects with properties (brand, model) and methods (start_engine).
- This approach is modular and helps in organizing code for large projects.
What Should You Choose—Functional Programming vs OOP?
If you prefer a structured, modular design, OOP is better. If you want small, independent functions that don’t modify state, FP is your way to go.
2. State Management
FP (Functional Programming)
FP has an immutable state, meaning that instead of modifying existing data, a new version of the data is created, unlike OOP.
Example of Immutable State in FP:
def deposit(balance, amount):
return balance + amount
new_balance = deposit(1000, 500) # Returns 1500
print(new_balance)
- The original balance remains unchanged, ensuring better predictability and security in concurrent applications.
OOP (Object-Oriented Programming)
In OOP, objects have mutable state, meaning their data (attributes) can change during the execution of the program over time.
Example of Mutable State in OOP:
class BankAccount:
def __init__(self, balance):
self.balance = balance
def deposit(self, amount):
self.balance += amount
account = BankAccount(1000)
account.deposit(500) # Now balance is 1500
print(account.balance)
- The balance of the BankAccount object changes when we deposit money.
- This approach is useful for applications where state changes are required, such as banking systems and inventory management.
What Should You Choose—Functional Programming vs OOP?
If your application requires constant updates and state changes, OOP is more intuitive. But if data consistency and immutability are important (e.g., financial transactions, multi-threaded apps), FP is a safer choice.
3. Code Reusability and Modularity
FP (Functional Programming)
In FP, codes are reused with high-order functions. They are treated as first-class citizens, meaning they can be passed as arguments or returned from other functions.
Example of Higher-Order Function in FP:
def apply_function(func, value):
return func(value)
def double(num):
return num * 2
print(apply_function(double, 5)) # Output: 10
- Functions are modular and reusable, making FP great for mathematical computations and data transformations.
OOP (Object-Oriented Programming)
OOP allows code reuse using inheritance and polymorphism, where child classes inherit properties and methods from parent classes.
Example of Inheritance in OOP:
class Animal:
def speak(self):
return "This animal makes a sound."
class Dog(Animal):
def speak(self):
return "Woof!"
dog = Dog()
print(dog.speak()) # Output: Woof!
- The Dog class inherits from Animal but overrides the speak method.
- This is useful for large-scale applications where multiple components share common behavior.
When Should Your Choose—Functional Programming vs OOP?
If you want hierarchical code organization, OOP is a better fit. But if you prefer independent, reusable functions, FP is more efficient.
4. Concurrency and Parallel Processing
FP (Functional Programming)
Since FP uses immutable data and pure functions, it’s ideal for parallel execution and optimized for multi-threading.
OOP (Object-Oriented Programming)
On the other hand, since OOP modifies shared state, multi-threading can cause issues if multiple objects modify the same data. Therefore, it is harder to handle concurrency in OOP.
What Should You Choose—Functional Programming vs OOP?
FP is more suitable for multi-threaded applications, AI, and real-time data processing, while OOP works well for single-threaded applications like desktop apps.
5. Security and Bug Prevention
FP (Functional Programming)
Since FP avoids modifying data, it reduces unintended side effects and security vulnerabilities. It’s more secure due to its immutable feature. This is why FP is preferred in high-security domains like banking, cryptography, and blockchain.
OOP (Object-Oriented Programming)
If an object’s state is accidentally modified, it can lead to unexpected behavior and hard-to-debug errors. Therefore, OOP are more prone to bugs due to state changes. For example, an unauthorized change in a banking system’s balance could cause major financial discrepancies.
What Should You Choose—Functional Programming vs OOP?
FP is inherently more secure due to its immutable nature, making it better for finance, healthcare, and cybersecurity applications. Whereas, OOP is ideal for game development, ERP, CRM, and UI-driven applications.
Want to build flawless frontend? Blend the magic of OOP and functional programming with experts!
To Wrap Up
The difference between functional programming vs OOP boils down to the type of application you’re building:
- If you need structure, real-world modeling with changing states—> OOP is better.
- If you need scalability, concurrency, and security—> FP is the smarter choice.
- Many modern languages support both (Python, JavaScript, Scala)—> The best approach is often a hybrid model.
Lastly, we must understand that the debate between functional programming vs OOP isn’t about choosing a winner; it’s about selecting the right approach for your project. And when we talk about modern development, it’s about blending both. Many languages like Python, JavaScript, and Scala allow you to leverage the best of both.
Therefore, the smartest approach is to choose the one or mix both that aligns with your project’s complexity, performance needs, and long-term scalability. If you’re still confused or want to learn more about it with regard to your project, it’s better to take an expert’s point of view.
Pixel-perfect, high-performance frontends start here!
Frequently Asked Questions
1. In functional programming vs OOP, how is OOP different from FP?
In functional programming vs OOP, OOP organizes code into objects with changing states, which makes it ideal for structured applications. Whereas, functional programming focuses on pure functions and immutability, ensuring better scalability and performance in data-heavy tasks.
2. Is Python a functional or OOP?
Python supports both. It allows OOP with classes and objects while enabling functional programming with first-class functions and immutability.
3. Is JavaScript OOP or FP?
JavaScript is a hybrid programming language. It supports OOP with prototypes and classes while allowing functional programming with higher-order functions and closures.
4. What is an example of functional programming?
Some of the examples of functional programming include Apache Spark (used in data analytics), TensorFlow (used in AI), and Quantitative Trading Systems (used in finance).
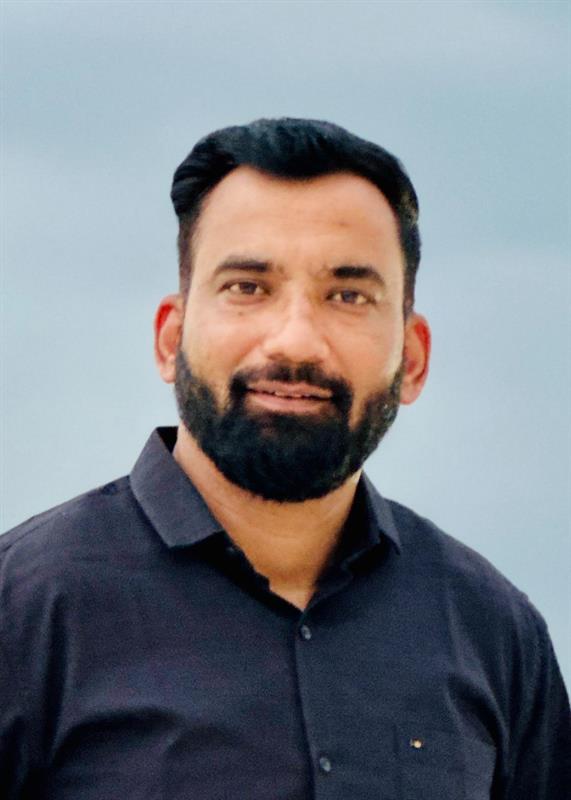
Excellence-driven professional with 15+ years of experience in increasing productivity, and revenue, while effectively managing products of all sizes. He has worked for international clients in the US, UK, and Singapore and local companies in various domains. With excellent attention to detail and a methodical approach to execution, he is an expert in bringing projects to a successful stage. He follows James Humes’s famous saying- “The art of communication is the language of leadership.”